-
EcmaScript 2017 .getOwnProperty and trailing commas
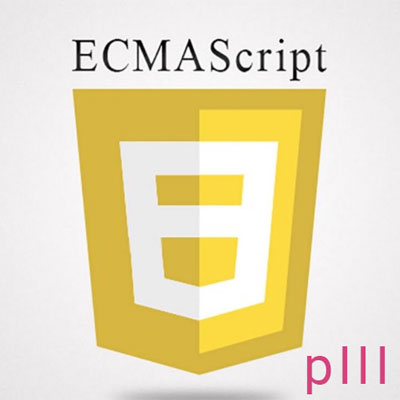
Continuing the series of our articles, - "EcmaScript 2017. Object.values/Object.entries" and "EcmaScript 2017. String padding", about EsmaScript 8 and its latest novelties, here we want to go through such features as: Object.getOwnPropertyDescriptors by Jordan Harband and Andrea Giammarchi, and trailing commas in function parameter lists and calls by Jeff Morrison.
Object.getOwnPropertyDescriptors
All of the own properties descriptors of the given object are being returned by the getOwnPropertyDescriptors method. It provides an opportunity to examine the precise description of all own properties of an object. A string-valued name and a property descriptor comprise a property in JavaScript.
So, long story short, this function is about retrieving in one single operation all possible own descriptors of a generic object. To simplify some common boilerplate and be consistent with the fact that there is a singular version of the method is the main goal.
obj
is the object for which to get all own property descriptors. It's the source object.
An object containing all own property descriptors of an object is the return value. If there are no properties it might be an empty object.
Defined directly on the object an own property descriptor is not inherited from the object's prototype.
Syntax of the function is:
Object.getOwnPropertyDescriptors(obj)
Attributes of a property descriptor:
value
is the value associated with the property (data descriptors only).
writable
- true
if and only if the value associated with the property may be changed (data descriptors only).
get
is a function which serves as a getter for the property, or undefined
if there is no getter (accessor descriptors only).
set
is a function which serves as a setter for the property, or undefined
if there is no setter (accessor descriptors only).
configurable
- true
if and only if the type of this property descriptor may be changed and if the property may be deleted from the corresponding object.
enumerable
- true
if and only if this property shows up during enumeration of the properties on the corresponding object.
Example:
const obj = { get es7() { return 777; }, get es8() { return 888; } }; Object.getOwnPropertyDescriptors(obj); // { // es7: { // configurable: true, // enumerable: true, // get: function es7(){}, //the getter function // set: undefined // }, // es8: { // configurable: true, // enumerable: true, // get: function es8(){}, //the getter function // set: undefined // } // }
Browser support (MDN) for Object.getOwnPropertyDescriptors:
Chrome - | 54 |
Firefox (Gecko) - | 50 (50) |
Edge - | (Yes) |
Internet Explorer - | ? |
Opera - | 41 |
Safari - | 10 |
Trailing commas in function parameter lists and calls
When you're adding new elements, parameters, or properties to JavaScript code, trailing commas can be very useful. It's sometimes called "final commas". You can simply add a new line, when adding a new property, without modifying the previously ending line if it already uses a trailing comma. As a result the editing code might be less tricky and version-control diffs cleaner.
Trailing commas has been allowed by JavaScript in array literals since the beginning. Later they were added to object literals in ECMAScript 5 and only most recently to function parameters in ES 8.
Example of trailing commas in object literals:
let obj = { first: 'Jane', last: 'Doe', };
and in Array literals:
let arr = [ 'red', 'green', 'blue', ]; console.log(arr.length); // 3
So, the ability of the compiler not to raise an error (SyntaxError
) when unnecessary comma was added in the end of the list is called trailing commas in function parameter.
function es8(var1, var2, var3,) { // ... }
This can be applied on function's calls:
es8(10, 20, 30,);
The benefits of this function are that, first of all, it's simpler to rearrange items, because there's no need to add and remove commas if the last item changes its position, and, second of all, it helps version control systems with tracking what actually changed.
For example, going from:
[ 'foo' ]
to:
[ 'foo', 'bar' ]
leads to both the lines with 'foo' and with 'bar' are being marked as changed, even though the only real change is the addition of the last line.
So, this feature was inspired from the trailing of commas in object literals and Array literals [10, 20, 30,]
and {x: 1,}
.
Wrapping up
In this article we've reviewed another two of the new features of EcmaScript 8 – Object.getOwnPropertyDescriptors and trailing commas in function parameter lists and calls. We sincerely hope that the publication was useful for you and if so, please, follow us for the next thing coming.
Welcome to CheckiO - games for coders where you can improve your codings skills.
The main idea behind these games is to give you the opportunity to learn by exchanging experience with the rest of the community. Every day we are trying to find interesting solutions for you to help you become a better coder.
Join the Game