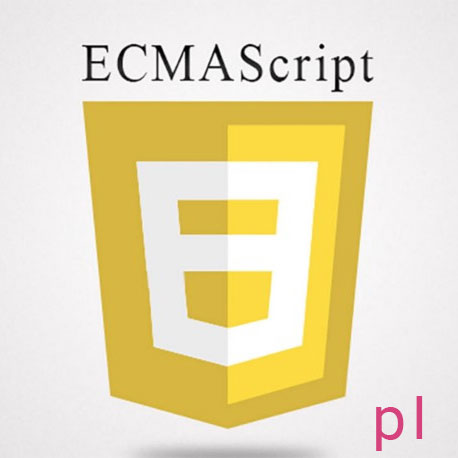
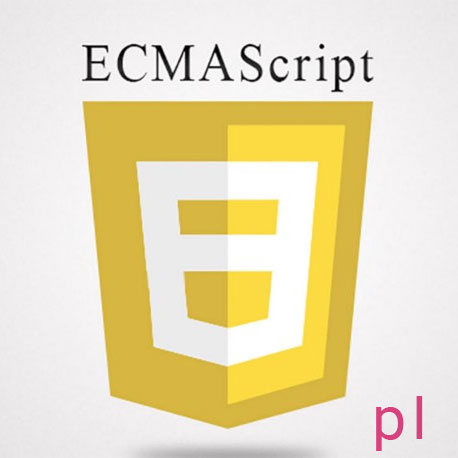
TC39 officially released EcmaScript 8 or EcmaScript 2017 at the end of June. The standard by now has been to publish a new ES specification version once a year. And because we follow the changes in the development of JavaScript we decided to start a series of articles with the latest innovations.
To simply list the final feature set of ES8 it consists of the major new features as: async functions and shared memory and atomics, and - the minor new features like: Object.values/Object.entries, string padding, Object.getOwnPropertyDescriptors, and trailing commas in function parameter lists and calls.
In this article we want to concentrate our attention on one of those minor new features, Object.values/Object.entries, to be precise.
Object.values
An array of values of the enumerated object's own properties in the Object.values method are being returned in the same order as provided by a for in loop.
So this method returns an array whose elements are the enumerable property values found on the object.
The declaration of the function looks like this:
Object.values(obj)
The obj
parameter is the object whose enumerable own property values are to be returned, it's the source for the operation.
An array that contains the object's enumerable own property values is the return value.
const obj = { a: 'aaa', b: 1, c: 'C' }; Object.values(obj); // ['aaa', 1, 'C'] const obj = ['c', 'i', 'o']; // same as { 0: 'c', 1: 'i', 2: 'o' }; Object.values(obj); // ['c', 'i', 'o'] // when we use numeric keys, the values returned in a numerical // order according to the keys const obj = { 10: 'aaa', 1: 'bbb', 5: 'ccc' }; Object.values(obj); // ['bbb', 'ccc', 'aaa'] Object.values('checkio'); // ['c', 'h', 'e', 'c', 'k', 'i', 'o']
Browser support (MDN) for Object.values:
Chrome - | 54 |
Edge - | (yes) |
Firefox (Gecko) - | 47 (47) |
Internet Explorer - | No support |
Opera - | No support |
Safari - | No support |
Object.entries
In the Object.entries method an array of object's enumerable own property [key, value] pairs are being returned in the same order as the Object.values.
So an array whose elements are arrays that correspond to enumerable property [key, value] pairs found directly upon the object are being returned by this method. The order of properties is the same as that of looping over the object's properties manually.
Syntax for this function is:
Object.entries(obj)
The obj
parameter is the object whose enumerable own property [key, value] pairs are to be returned.
An array that contains the object's own enumerable property [key, value] pairs is the return value.
const obj = { a: 'aaa', b: 1, c: 'C' }; Object.entries(obj); // [['a', 'aaa], ['b', 1], ['c', 'C']] // It's important to point out that JavaScript has string-keyed properties, // and that’s why '0', '1' and '2' are strings. const obj = ['c', 'i', 'o']; Object.entries(obj); // [['0', 'c'], ['1', 'i'], ['2', 'o']] const obj = { 10: 'aaa', 1: 'bbb', 5: 'ccc' }; Object.entries(obj); // [['1', 'bbb'], ['5', 'ccc'], ['10', 'aaa']] Object.entries('cio'); // [['0', 'c'], ['1', 'i'], ['2', 'o']]
Browser support (MDN) for Object.entries:
Chrome - | 54 |
Edge - | (yes) |
Firefox (Gecko) - | 47 (47) |
Internet Explorer - | No support |
Opera - | No support |
Safari - | 10.1 |
Examples from CheckiO
You can also check out some of the solutions from our users:
A bit crEATive - here we can see a creative solution from a user asortfixing, where he is simply showing that Object.values returns the same list as it was given.
More clear solution - from franck.diedrich in mission The Most Wanted Letter - the function Object.values was used to get the maximum value of a given object using this code:
const max = Math.max.apply(null, Object.values(occ))
Interesting. Here is an example of using the function Object.entries from a member ostroffskiy where the maximum key was founded using the following code:
const res = Object.entries(counter).sort().reduce((old, current) => { if (old[1] < current[1]) { return current } return old; }, ['a',0]); return res[0];
Most Wanted Latter - almost the same version for the same mission, but a memeber AnyKey111 wasn't using a reduce function this time.
Wrapping things up
In this article we reviewed only one of the new features of EcmaScript 8 - Object.values/Object.entries. If this topic was useful for you and you want to find out more, then you are welcome to follow our further publications. In the next articles we are going to talk about the ES8 other features, such as: string padding and trailing commas in function parameter lists and calls.