-
EcmaScript 2017. String padding
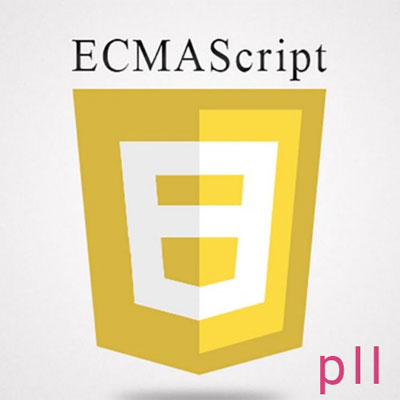
As some of you, who have been following our posts, might remember, in the previous article we've reviewed one of the latest EcmaScript 2007's features - Object.values/Object.entries. And as we've promised, we're continuing talking about the EsmaScript's innovations and now we want to have a closer look on the other new feature called String padding which was proposed by Jordan Harband and Rick Waldron.
String methods provide the creation of a new string. It consists of an original string padded with leading or trailing characters to a specified total length. The padding could be done with a specified character or string or just with spaces by default.
Padding strings is generally used in such cases as when you're trying to display tabular data in a monospaced font or align a console output ('Test 001: ✔'
), print hexadecimal or binary numbers that have a fixed number of digits ('0x00FF'
) or add a count or an ID to a file name or a URL ('file 001.txt'
).
String padding section adds two functions to the String object: padStart and padEnd. Without them language feels incomplete. They are commonly used and exist in a majority of websites and frameworks. The web performance and developer’s productivity will be improved by bringing this into the platform, because the majority of current implementations of string padding are inefficient.
String.prototype.padStart
The current string is being padded (repeatedly, if needed) with another string in the padStart()
method so that the resulting string reached the given length. The padding is applied from the start of the current string.
The function's syntax:
str.padStart(targetLength [, padString])
Describing it simply, to the start of a string by .padStart
will be added enough spaces (repeated fillString) so that it arrived at the requested length.
When the specified max length of a string is reached, no padding characters will be inserted.
abc'.padStart(3); // <- 'abc' 'abc'.padStart(2); // <- 'abc'
The padding character could be changed to something else, other than a space.
'abc'.padStart(5, 'x'); // <- 'xxabc'
But it doesn't have to be a single character. If anything, to meet the character length requirement the padding string will be sliced.
'abc'.padStart(7, 'xo'); // <- 'xoxoabc' 'abc'.padStart(6, 'xo'); // <- 'xoxabc'
The fillString
becomes a mask into which the receiver is inserted at the end, if maxLength
and fillString.length
are the same.
> 'abc'.padStart(10, '0123456789') '0123456abc'
A simple example of how the .padStart
works:
String.prototype.padStart = function (maxLength, fillString=' ') { let str = String(this); if (str.length >= maxLength) { return str; } fillString = String(fillString); if (fillString.length === 0) { fillString = ' '; } let fillLen = maxLength - str.length; let timesToRepeat = Math.ceil(fillLen / fillString.length); let truncatedStringFiller = fillString .repeat(timesToRepeat) .slice(0, fillLen); return truncatedStringFiller + str; };
String.prototype.padEnd
The current string is being padded (repeatedly, if needed) with another string in the padEnd()
method so that the resulting string reached the given length. The padding is applied from the end of the current string.
The function's syntax:
str.padEnd(targetLength [, padString])
Simply put, the .padEnd
method works similarly to the .padStart
method with the one difference being that the padding (inserting repeated fillString
) is appended to the string.
123'.padEnd(6); // <- '123 '
The padding character can also be specified.
123'.padEnd(6, 'x'); // <- '123xxx'
A simple example of .padEnd
:
> 'x'.padEnd(5, 'ab') 'xabab' > 'x'.padEnd(4, 'ab') 'xaba' > 'abcd'.padEnd(2, '#') 'abcd' > 'abc'.padEnd(10, '0123456789') 'abc0123456' > 'x'.padEnd(3) 'x ' return str + truncatedStringFiller;
So, as you can see, those functions have two parameters. The first one is the targetLength and it's the total length of the resulted string. The optional padString is the second parameter and it's the string to pad the source string. Space here is being the default value.
es8'.padStart(2); // 'es8' 'es8'.padStart(5); // ' es8' 'es8'.padStart(6, 'woof'); // 'wooes8' 'es8'.padStart(14, 'wow'); // 'wowwowwowwoes8' 'es8'.padStart(7, '0'); // '0000es8' 'es8'.padEnd(2); // 'es8' 'es8'.padEnd(5); // 'es8 ' 'es8'.padEnd(6, 'woof'); // 'es8woo' 'es8'.padEnd(14, 'wow'); // 'es8wowwowwowwo' 'es8'.padEnd(7, '6'); // 'es86666'
Browser support (MDN) for String padding:
Chrome - | 57 |
Firefox - | 48 |
Edge - | 15 |
Internet Explorer - | 15 |
Opera - | 44 |
Safari - | 10 |
So, as you might noticed, we all already have been using these functions in libraries, like Lodash.
For example, the function padStart
:
_.padEnd('abc', 6); // => 'abc ' _.padEnd('abc', 6, '_-'); // => 'abc_-_' _.padEnd('abc', 3); // => 'abc'and the function
padEnd
:
_.padStart('abc', 6); // => ' abc' _.padStart('abc', 6, '_-'); // => '_-_abc' _.padStart('abc', 3); // => 'abc'
Interesting thing is that the terms left and right for the bidirectional or left-to-right languages don't work very well, that's why the padding methods are called padStart
and padEnd
.
padStart in CheckiO
You can check out one of the CheckiO solutions of the "Hamming Distance" mission, where it was important to have same size strings to compare in iteration. This is where padStart
was so useful:
n = n.toString(2).padStart(32, '0')
Conclusion
In this article we've continued reviewing another one of the new features of EcmaScript 8 – String padding. If you like this information then stay with us for the next publication where we'll go through such features as: Object.getOwnPropertyDescriptors and trailing commas in function parameter lists and calls.
Welcome to CheckiO - games for coders where you can improve your codings skills.
The main idea behind these games is to give you the opportunity to learn by exchanging experience with the rest of the community. Every day we are trying to find interesting solutions for you to help you become a better coder.
Join the Game