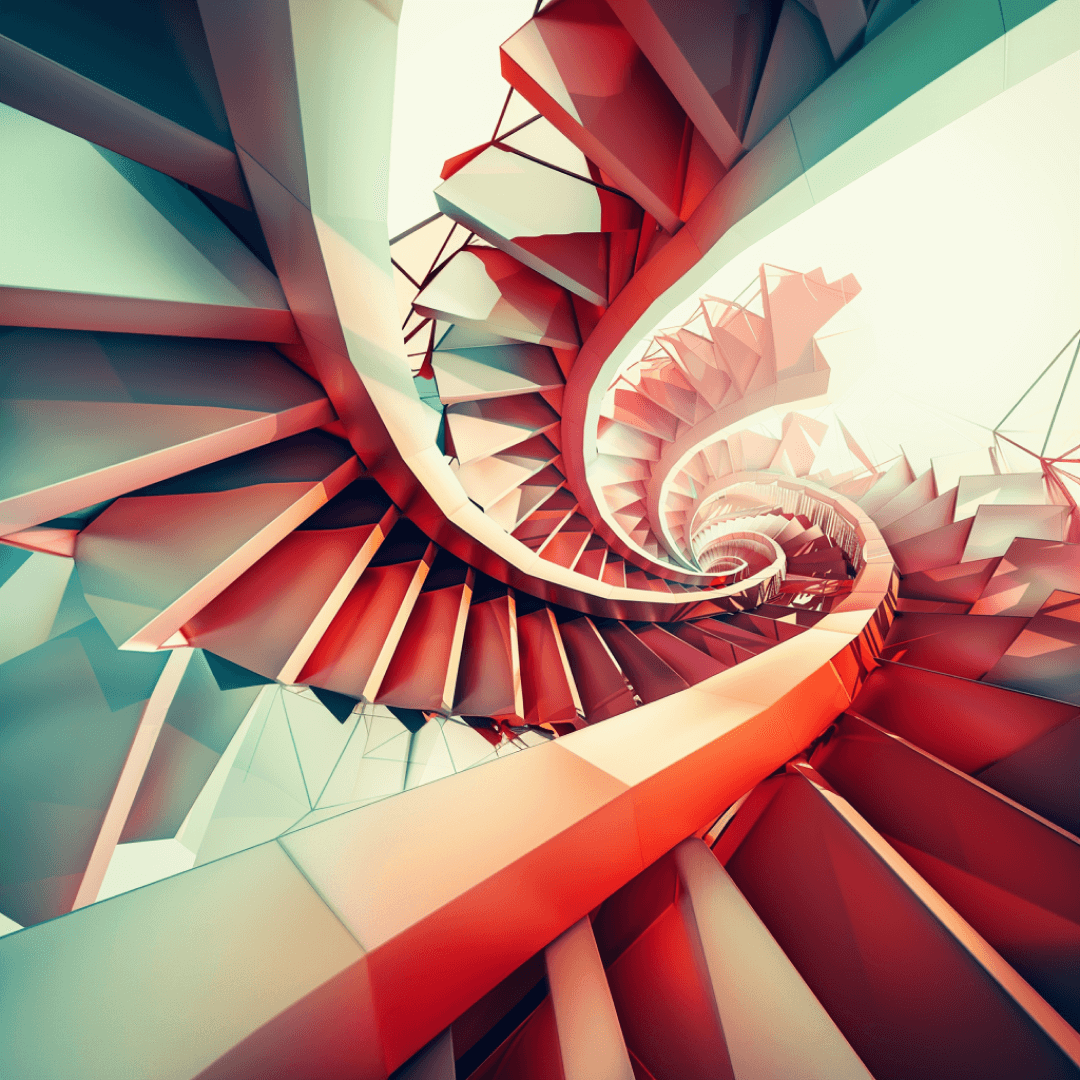
Hello, checkiomates🐱👤!
In this edition, we dive into JavaScript’s evolving landscape. Explore insights on the Nullish Coalescing Assignment Operator, a tool for cleaner and more intuitive code. Learn about innovative component design patterns with Component Party. Finally, uncover the practical value of generator functions, breaking down their unique power for managing complex data flows.
💡TIP
At your profile, after clicking on big percent number of your progress, you may see module and methods you have already used in your shared solutions. If you want to discover all CheckiO features, visit our tutorial. It's a longread, but it's worth it!
🏁MISSION
Staircase by freeman_lex -
The easier problem Beat the previous asked you to greedily extract a strictly ascending sequence of integers from the given series of digits. For example, for digits equal to "31415926", the array of integers returned should be [3, 14, 15, 92], with the last original digit left unused. Going somewhat against intuition, the ability to tactically skip some of the digits at will may allow the resulting array of integers to contain more elements than the list constructed in the previous greedy fashion. With this additional freedom, the example digit string "31415926" would allow the result [3, 4, 5, 9, 26] with one more element than the greedily constructed solution. Your function should return the length of the longest array of ascending integers that can be extracted from digits. Note that you are allowed to skip one or more digits not just between two integers being extracted, but during the construction of each such integer.
assert.strictEqual(staircase("100123"), 4); assert.strictEqual(staircase("503715"), 4); assert.strictEqual(staircase("0425494220946"), 6);
📖ARTICLE
A Rosetta Stone of UI Libraries -
A long-standing comparison of many different frameworks (like React, Vue, Svelte, Angular, Qwik, Solid.js, etc.) by way of simple code snippets to perform various tasks. Now including Svelte 5 and Angular 17/Renaissance.
JavaScript's ??= Operator: Default Values Made Simple -
The nullish coalescing assignment operator ??= is relatively new to JavaScript. It was officially added in ECMAScript 2021 (ES12) as part of the “Logical Assignment Operators” proposal. Think of ??= as a smart guardian for your variables. It only assigns a new value if the current one is null or undefined
Why would anyone need JavaScript generator functions? -
Generators are an odd part of the JavaScript language. And some people find them a bit of a puzzle. You might be a successful developer for decades and never feel the need to reach for them. Which raises the question, if you can go so long without ever needing them, what are they good for?
👩💻CODE SHOT
How do you think, what the following code does?
function ?????????(data: number[]): number[] { let biggest = -1 const results = [] for(let i = data.length - 1; i >= 0; i--){ results.push(biggest) biggest = Math.max(biggest, data[i]) } return results.reverse() }
🙌 Thanks for your attention! Hope to meet you at CheckiO, as well as at our Instagram and Twitter! We are really interested in your thoughts! Please, leave a comment below! ⤵