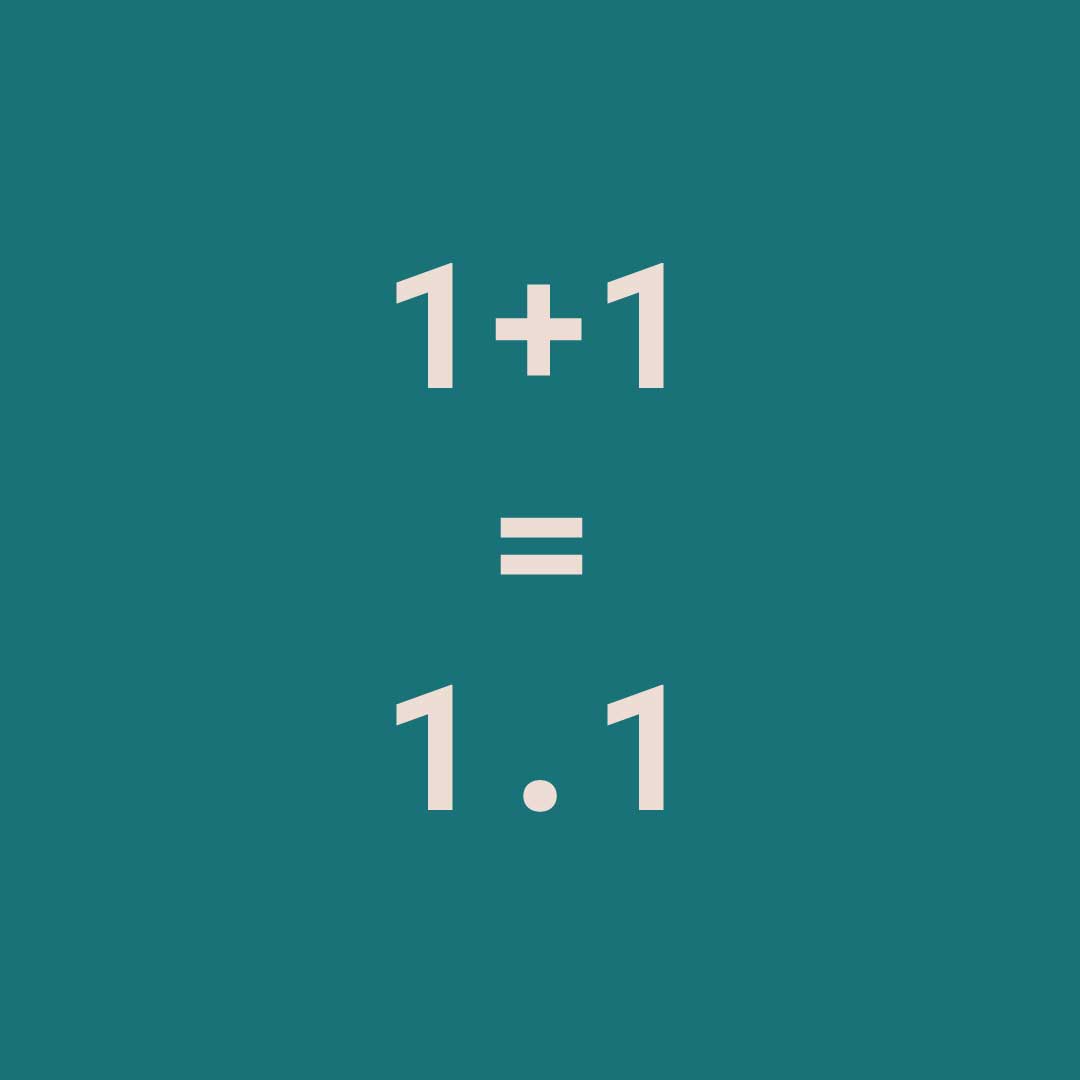
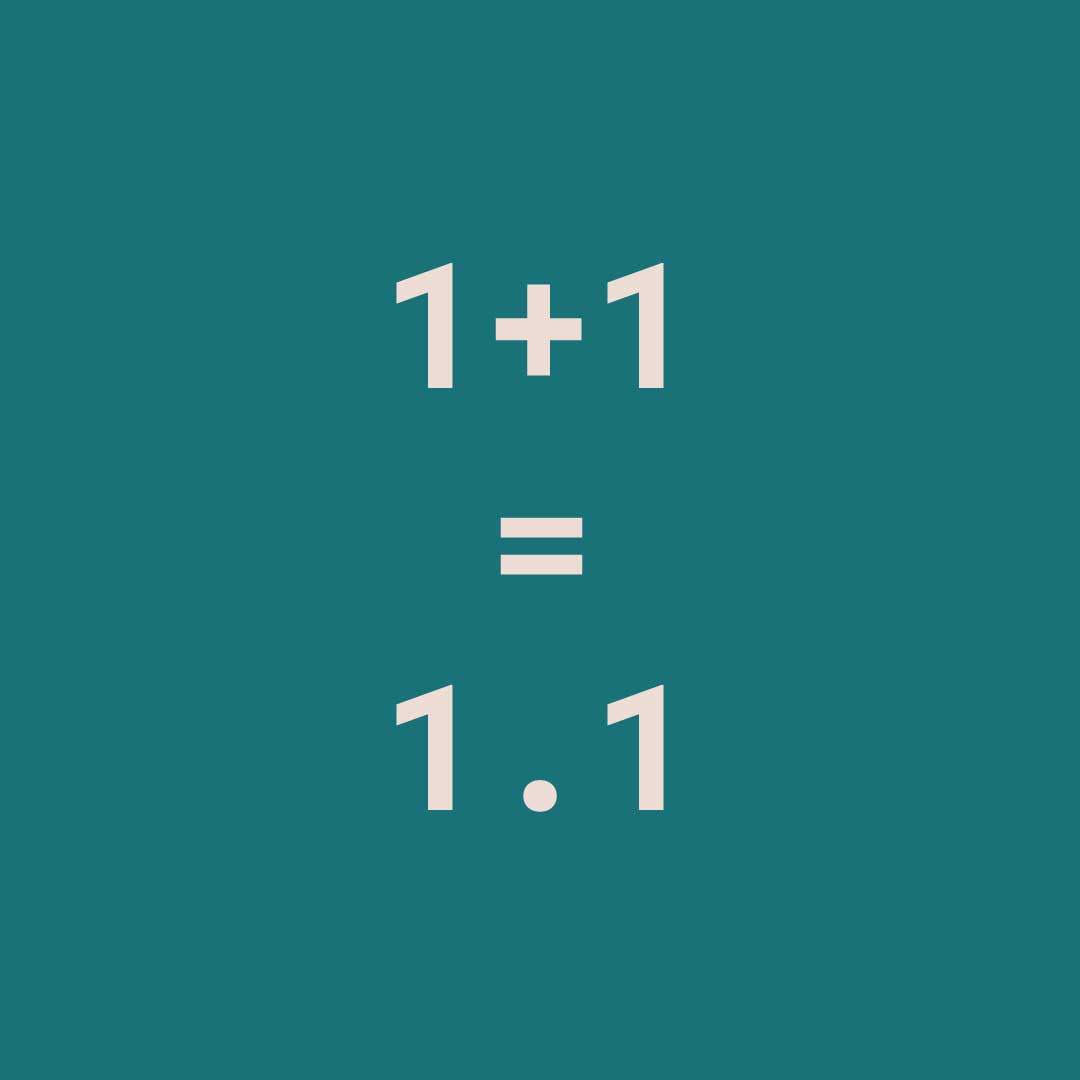
JavaScript is a client-side scripting language, very similar to other scripting languages and used in almost every web application. It's an easy one to get started with. But as you go along there're a lot of coding traps you can fall into. In CheckiO we often see the same mistakes are being made over and over again, so here I wanted to lay them out for everyone to recognize and take a point of not repeating ever again.
Mismatching quotes, parenthesis and curly brace
If you want to to avoid mismatched quotes, parentheses and curly brackets then you have to always code your opening and closing element at the same time, then step into the element to add your code, like this:
var myString = ""; //code the quotes before entering your string value function myFunction(){ if(){ //close out every bracket when you open one. } } //count all the left parens and right parens and make sure they're equal alert(parseInt(var1)*(parseInt(var2)+parseInt(var3))); //close every parenthesis when a parenthesis is open
If you've opened an element, you need to close it. Start putting the arguments for a function into the parenthesis after you've added the closing parenthesis. In case you have a bunch of parenthesis, count the opening parenthesis and the closing parenthesis, and make sure that those two numbers are equal.
Incorrect usage of 'if' statement comparison
Here I'm talking about the "=="" operator which does a comparison and the "=" operator which assigns a value to a variable. The error created depends on the language. Some languages will throw an error, but JavaScript will actually evaluate the statement and return true or false.
For example:
var x = 5; if (x == 5)
As expected it evaluates if x is equal to 5. And since the result is true, any statements within the 'if' clause are executed.
But when the developer makes a typo:
var x = 0; if (x = 5)
Now instead of using the comparison operator, the developer accidentally used the assignment operator. In some languages, this would throw an error, but in JavaScript the result won't be evaluated to false, but instead, it evaluates to true.
Another common typo:
var x = 5; if (x = 1)
In Boolean notation, 1 is true and 0 is false. You might expect the result of this statement to be false, but since 1 is equal to true, the result is true.
Testing can caught this type of logic error, but always ensure that you're using the comparison operator with your 'if' statements.
No block-level scope
In many languages, you can define a variable just for your loop structure. You loop through a variable and it's destroyed once the loop exists.
Just look at the following code:
for (var j = 0; j < 11; j++) { j = j + 1; } console.log ( j );
Most experienced developers would think that in the code above "j" would be null. The j variable can be used in the "for" loop in other languages, but it's destroyed after the loop is finished.
But in JavaScript the output for the j variable would be 10. This can cause serious bugs in your code. It's easy to miss this language quirk for new JavaScript developers.
Using named objects indexes as arrays
Arrays in JavaScript use numeric integer indexes. It's also possible to use objects similarly to arrays, but objects use named indexes. If you attempt to use a named index in an array, your code will return the wrong result.
var color = []; color[0] = "blue"; color[1] = "purple"; color[2] = “orange”; var x = color.length; var y = color [0];As you can see an array named "color" is created. We assign colors to the first three indexes, then evaluate the length and assign the first color to the variable y. The variable x evaluates to 3 and the variable y contains the value "blue". This is typical behavior for an array.
Let's create an object named car:
var car = []; car ["color"] = "red"; car ["make"] = "chevy"; car ["model"] = “corvette”; var x = car.length; var y = car [0];
The code above defines an object. Because of the named indexes you know that it's an object rather than an array. The car has three named indexes that define it. The issue is with the x and y variables. These values, unlike the array, are undefined and don't evaluate to valid results. The length property evaluates to 0 and y is assigned "undefined".
Working with these two data types, make sure you know which one you're dealing with before you use certain calculations and properties.
Confusing undefined and null
In other languages, you can set null to variables. JavaScript uses undefined and null, but it defaults variables to undefined and objects to null. These values are assigned when an incorrect calculation is made or you assign a null reference to an object. For an object to be null, it must be defined, so you must be careful when you compare objects.
The following code will give you an error if the object is undefined:
if (object !== null && typeof object !== "undefined")
Always check if the object is undefined first, then identify if it's null.
if (typeof object !== "undefined" && object!== null)
Confusing addition and concatenation
Addition is about adding numbers and concatenation is about adding strings. In JavaScript both operations use the same + operator. Because of this, adding a number as a number will produce a different result from adding a number as a string:
var x = 10 + 5; // the result in x is 15 var x = 10 + "5"; // the result in x is "105"
When adding two variables, it can be difficult to anticipate the result:
var x = 10; var y = 5; var z = x + y; // the result in z is 15 var x = 10; var y = "5"; var z = x + y; // the result in z is "105"
Breaking a return statement
JavaScript closes a statement automatically at the end of a line. Because of this, these two examples will return the same result:
function myFunction(a) { var power = 10 return a * power }
function myFunction(a) { var power = 10; return a * power; }
JavaScript will also allow you to break a statement into two lines, and that's why the next example will also return the same result:
function myFunction(a) { var power = 10; return a * power; }
But, what will happen if you break the return statement in two lines like this:
function myFunction(a) { var power = 10; return a * power; }
Well, the function will return undefined. Because JavaScript thinks you meant:
function myFunction(a) { var power = 10; return; a * power; }
If a statement is incomplete like:
var
JavaScript will try to complete the statement by reading the next line:
power = 10;
But since this statement is complete:
return
JavaScript will automatically close it like this:
return;
This happens because closing/ending statements with semicolon is optional in JavaScript. JavaScript will close the return statement at the end of the line, because it's a complete statement.
Overwriting functions / overloading functions
When you declare a function more than once, the last declaration of that function will overwrite all previous version of that function throwing no errors or warnings. This is different from other programming languages, where you can have multiple functions with the same name as long as they take different arguments. But there is no overloading in JavaScript. This makes it very important not to use the names of core JavaScript functions in your code. Also, watch it when including multiple JavaScript files, as an included script may overwrite a function in another script. Use anonymous functions and namespaces.
(function(){ // creation of my namespace // if namespace doesn't exist, create it. if(!window.MYNAMESPACE) { window['MYNAMESPACE'] = {}; } // this function only accessible within the anonymous function function myFunction(var1, var2){ //local function code goes here } /* attaches the local function to the namespace making it accessible outside of the anoymous function with use of namespace */ window['MYNAMESPACE']['myFunction'] = myFunction; })();// the parenthesis = immediate execution // parenthesis encompassing all the code make the function anonymous
Missing Parameters
A common error is forgetting to update all the function calls when you add a parameter to a function. If you need to add a parameter to handle a special case call in your function that you've already been calling, set a default value for that parameter in your function, just in case you missed updating one of the calls in one of your scripts.
function addressFunction(address, city, state, country){ country = country || "US"; // if country is not passed, assume USA //rest of code }
'this'
Another common mistake is forgetting to use 'this'. Functions defined on a JavaScript object accessing properties on that JavaScript object and failing to use the 'this' reference identifier. For example, the following is incorrect:
function myFunction() { var myObject = { objProperty: "some text", objMethod: function() { alert(objProperty); } }; myObject.objMethod(); }
function myFunction() { var myObject = { objProperty: "some text", objMethod: function() { alert(this.objProperty); } }; myObject.objMethod(); }
Conclusion
JavaScript is great and anyone who knows C, Java, or C# will pick up on its syntax easily as it's a C-style language. But JavaScript has several quirks that make it completely different than other languages in much of the way it handles statements, variables, and objects. So keep these mistakes in mind and it'll help you to avoid a lot of unnecessary problems. And what mistakes have you faced that I've failed to mention?